AppConnect
iOS and Android
AppConnect by PaySimple is an application for iOS and Android that enables easy payment collection from your app without the burden of PCI compliance.
AppConnect uses custom url schemes to transfer payment and customer data from your app.
If you are interested in integrating PaySimple AppConnect into your app, please contact our API Support Team at [email protected].
Launching PaySimple
The request you send will be url-encoded json sent as a query parameter value.
In order to initiate a payment with PaySimple you need to create a url and open it.
• Scheme: paysimple-v1://
• Host: make-payment
• Query: data
paysimple-v1://make-payment/?data=
Using the following JSON:
{
"CallbackUrl": "yourappname://payment-complete",
"AppName": "YourAppName",
"Customer": {
"FirstName": "John",
"LastName": "Doe",
},
"Total": 10.99,
"Description": "tree trimming"
}
The following url can be generated:
paysimple://make-payment/?data=%7B%22CallbackUrl%22%3A%22yourappname%3A%2F%2Fpayment-
complete%22%2C%22AppName%22%3A%22YourAppName%22%2C%22Customer%22%3A%7B
%22FirstName%22%3A%22Jeremy%22%2C%22LastName%22%3A%22Stevens%22%7D%2
C%22Total%22%3A10.99%2C%22Description%22%3A%22tree+trimming%22%7D
Once the url is created it just needs to be opened, this will then open the PaySimple application.
Completing the Payment
In order to finish the payment, PaySimple will return a response using a custom url scheme that your app registers and provides as a callback url in the request. The data will be a json url- encoded value of a query called data.
iOS
For iOS you need to add the CFBundleURLTypes to your Info.plist file such as:
<key>CFBundleURLTypes</key>
<array>
<dict>
<key>CFBundleTypeRole</key>
<string>Viewer</string>
<key>CFBundleURLName</key>
<string>com.yourappname.paymentcompleted</string>
<key>CFBundleURLSchemes</key>
<array>
<string>yourappname</string>
</array>
</dict>
</array>
You will also need to add the LSApplicationQueriesSchemes to your info.plist file exactly like this.
<key>LSApplicationQueriesSchemes</key>
<array>
<string>paysimple-v1</string>
</array>
You may also want to reference Apple’s documentation for custom url schemes.
Android
For Android you need to add an intent filter to your manifest file such as:
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<data android:scheme="yourappname"
android:host="payment-complete"/>
</intent-filter>
You may also want to reference Android’s documentation for deep linking.
Data Schema
Request Data
AppName | Type | Required | Description |
---|---|---|---|
AppName | String | Yes | The name of your application. This will just be used to display to your users |
CallbackUrl | String | Yes | This is the url that PaySimple will use to append the payment response to and return control back to your app. |
Customer | Customer Object | No | Customer information that will be tied to the payment. |
Description | String | No | This is an optional field that can be used for anything. It has a max length of 2048 characters. |
Total | Decimal | Yes | Possible Values: 0.01<Value<1999999.00 The total amount to bill the customer including taxes and fees. |
ForceLogout | Bool | No | Defaults to False. If passed in as True then this will force the AppConnect application to logout on load, requiring the user to log back in. Can be used in conjunction with partner app logout sequence. |
DisableCustomerAccess | Bool | No | Defaults to False. If passed in as True the user is not able to change the customer during checkout process. |
DisableAmountChange | Bool | No | Defaults to False. If passed in as True the user is not able to change the amount being charged during checkout process |
Customer
Property | Type | Required | Description |
---|---|---|---|
String | No | Email address for the customer. | |
FirstName | String | No | First name of the customer |
Id | Int | No | PaySimple existing customer id. If Id is passed, FirstName, LastName and Email will be treated as redundant since the CustomerID will be used to retrieve the account and that data will supersede all other customer data that was passed in. |
LastName | String | No | Last name of the customer. |
Response Data
Property | Type | Description |
---|---|---|
AmountPaid | Decimal | The actual amount that was paid |
CustomerId | Integer | The PaySimple id of the customer that was associated to the payment |
AccountId | Integer | The PaySimple id of the customer payment account that was created/used for the payment |
PaymentId | Integer | The id of the payment that can be used to retrieve details about the transaction from PaySimple |
PaymentType | PaymentType | The method of payment that was used |
PaymentSubType | String | Payment subtype, eg Visa, MasterCard for a CC payment or Checking, BusinessChecking, Savings for an ACH payment |
PurchaseOrderNumber | String | Original PO number that was passed in if present |
Status | PaymentStatus | The status of the payment transaction |
LastFour | String | Last four digits of the payment account |
ErrorMessage | String | Relevant messaging regarding Payment Failure reason, Customer Error or App Launch error |
PaymentStatus
Name | Description |
---|---|
Authorized | Initial status for Credit Card payments |
Failed | Payment failed. Retrieve payment for more details |
Posted | Initial status for ACH payments |
Cancelled | Transaction Cancelled before payment processing attempted |
PaymentType
Name | Description |
---|---|
ACH | A bank account |
CreditCard | A credit card |
Error Handling
Launching PaySimple App Error Handling
Error Type | Handling |
---|---|
Total is incorrectly formatted or not present | PaySimple will use CallbackUrl to launch partner app with Status of “Cancelled” and ErrorMessage |
Launch data is incorrectly formatted | PaySimple will use CallbackUrl to launch partner app with Status of “Cancelled” and ErrorMessage |
CallbackUrl is not present | If the CallbackUrl for the partner app is not present, PaySimple will launch normally as if no data was passed in. |
PaySimple app not found | In the event that the PaySimple app is not found the partner app is responsible for handling a redirect to the app store to download the PaySimple app. |
PaySimple in App Error Handling
Error Type | Handling |
---|---|
Customer Not Found | If customer.id is passed in and not found by PaySimple, the user will be presented with 2 options: 1. Continue with sale without the customer loaded. 2. Cancel the sale and return to the partner app with response data: - Status: Cancelled - AmountPaid: 0 - CustomerId: 0 |
Swipe or Key Entered payment failed | If a card or ACH account is declined or makes a failed payment for any reason the user will be presented with 3 options: 1. Enter another card/Ach manually (key enter) 2. Swipe another card 3. Cancel the sale and return to the partner app with response data: - Status: Failed - AmountPaid: 0 - CustomerId: CustomerId that was used or created - AccountId: AccountId that was used or created |
Mobile Swipe Truth Table
Scenarios
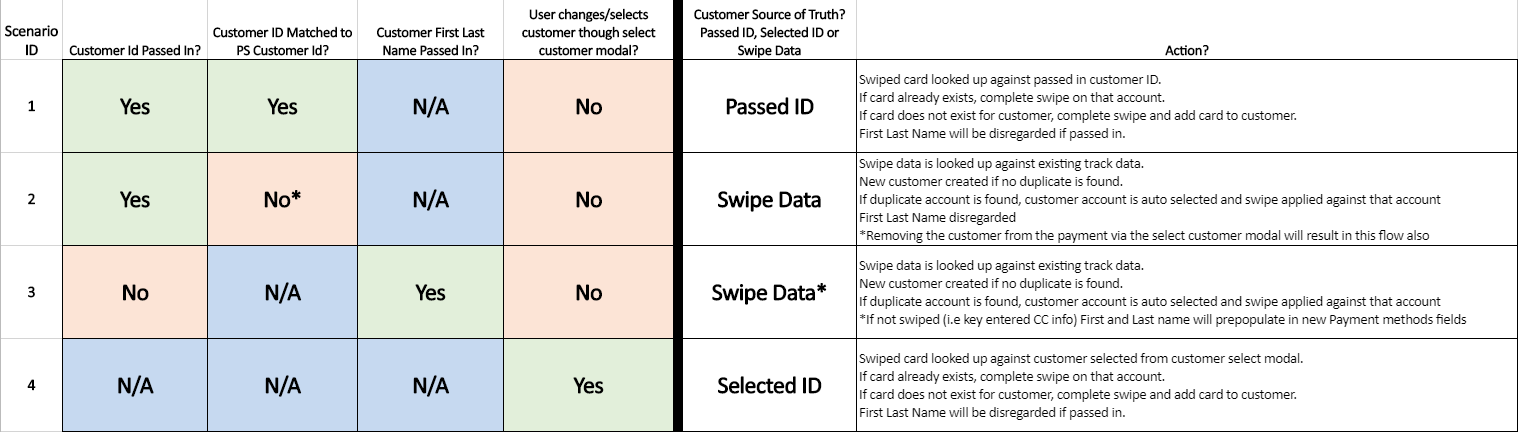
Updated about 1 month ago